Pop-up from Hell: On the growing opacity of web programs
I started to learn how to program in high school at the end of the 1990s using a mix of BASIC, Turbo Pascal and HTML with JavaScript. The seed for this blog post comes from my experience with learning how to program in JavaScript, without having much guidance or organized resources. This article continues a theme that I started in my interactive Commodore 64 article, which is to look at past programming systems and see what interesting past ideas have been lost in contemporary systems. Unlike with Commodore 64, which I first used in 2018 in the Seattle Living Computers museum, my perspective on the Early Web may be biased by personal experience. I will do my best to not make this post sound like a grumbling of an old nerd! (I thought this only comes later, but I may have been wrong...)
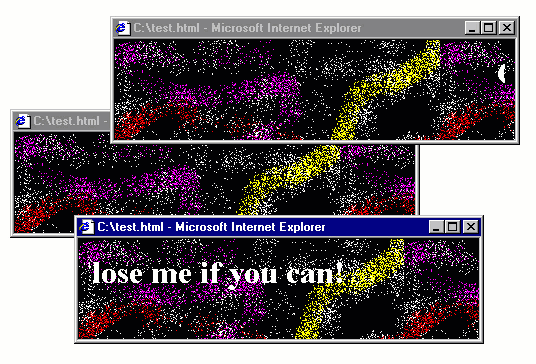
The 1990s, the web had a fair amount of quirky web pages, often created just for fun. The GeoCities hosting service, which has partly been archived is a witness of this and there are even academic books, such as Dot-Com Design documenting this history.
Some of the quirky things that you could do with JavaScript included creating roll-over effects (making an image change when mouse pointer is over it), creating an animation that follows the cursor as it moves and, of course, annoying the users with all sorts of pop-up windows for both entertaining and advertising purposes. Annoying pop-ups will be the starting point for my blog post, but I'll be using those to make a more general and interesting point about how programs evolve to become more opaque.
This blog post is based on a talk Popup from hell: Reflections on the most annoying 1990s program that I did recently at an (in person!) meeting of the PROGRAMme project. Thanks to everyone who attended for lively discussion and useful feedback!
Published: Friday, 8 October 2021, 1:14 PM
Tags:
academic, research, web, philosophy, talks
Read the complete article
Creating web sites with Suave: How to contribute to F# Snippets
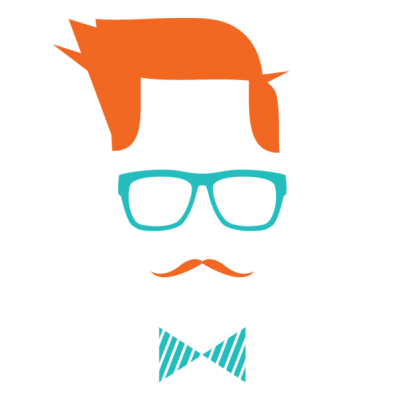
The core of many web sites and web APIs is very simple. Given an HTTP request,
produce a HTTP response. In F#, we can represent this as a function with type
Request -> Response
. To make our server scalable, we should make the function
asynchronous to avoid unnecessary blocking of threads. In F#, this can be
captured as Request -> Async<Response>
. Sounds pretty simple, right? So why
are there so many evil frameworks
that make simple web programming difficult?
Fortunately, there is a nice F# library called Suave.io that is based exactly on the above idea:
Suave is a simple web development F# library providing a lightweight web server and a set of combinators to manipulate route flow and task composition.
I recently decided to start a new version of the F# Snippets web site and I wanted to keep the implementation functional, simple, cross-platform and easy to contrbute to. I wrote a first prototype of the implementation using Suave and already received a few contributions via pull requests! In this blog post, I'll share a few interesting aspects of the implementation and I'll give you some good pointers where you can learn more about Suave. There is no excuse for not contributing to F# Snippets v2 after reading this blog post!
Published: Wednesday, 16 September 2015, 12:26 AM
Tags:
f#, web
Read the complete article
In the age of the web: Typed functional-first programming revisited
Most programming languages were designed before the age of web. This matters because the web changes many assumptions that typed functional language designers tak for granted. For example, programs do not run in a closed world, but must instead interact with (changing and likely unreliable) services and data sources, communication is often asynchronous or event-driven, and programs need to interoperate with untyped environments like JavaScript libraries.
How can statically-typed programming languages adapt to the modern world? In this article, I look at one possible answer that is inspired by the F# language and various F# libraries. In F#, we use type providers for integration with external information sources and for integration with untyped programming environments. We use lightweight meta-programming for targeting JavaScript and computation expressions for writing asynchronous code.
This blog post is a shorter version of a ML workshop paper that I co-authored earlier this year and you should see this more as a position statement. I'm not sure if F# and the solutions shown here are the best ones, but I think they highlight very important questions in programming language design that I very much see as unsolved.
The article has two sections. First, I'll go through a simple case study showing how F# can be used to build a client-side web widget. Then, I'll discuss some of the implications for programming language design based on the example.
Published: Wednesday, 9 September 2015, 6:14 PM
Tags:
f#, type providers, web, functional programming, research
Read the complete article
Real-World F# Articles on MSDN
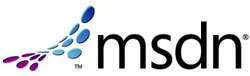
More than a year ago, Mike Stephens from Manning (who was also behind my Real-World Functional Programming book) asked me if I'd be interested in collaborating on a project for MSDN. The idea was to collaborate with Microsoft on creating some additional content for the official F# Documentation.
A few days ago, the new content appeared on MSDN, so I finally have an excuse for the recent lack of blogging! Although the content contains a large number of new articles that are not based on my book, you can find it in the MSDN section named after my book, right under Visual F#. If you can't wait to check it out, here are all the links:
- The Real-World Functional Programming section on MSDN.
- The source code for individual chapters is available on MSDN Code Gallery.
- I also published annotated TOC with source code links on my functional programming web site.
While working on the articles, I also wrote about a few topics that we didn't use in the final version. You'll see them on my blog in the next few days, as soon as I edit them into a blog post form. Continue reading for more information about individual chapters.
Published: Wednesday, 10 August 2011, 4:38 AM
Tags:
functional, web, asynchronous, parallel, links, f#, c#
Read the complete article
Dynamic in F#: Reading data from SQL database
When reading data from a SQL database in F#, you have a couple of options.
You can use F# implementation of LINQ, which allows you to write queries directly
in the F# language or you can use standard ADO.NET classes such as SqlCommand
.
The second option is often good enough - when you just need to call a simple
query, you probably don't want to setup the whole LINQ infrastructure (generate
classes, reference F# PowerPack, etc.) Unfortunately, ADO.NET classes are a bit
ugly to use. When calling an SQL stored procedure, you need to specify the name
of the procedure as a string, you need to add parameters one-by-one by creating
instances of SqlParameter
, you need to dynamically cast results from
the obj
type and so on...
In this article, we'll look how to use the dynamic operator to make the experience of using ADO.NET from F# dramatically better. Dynamic operator (there are actually two of them) are a simple way of supporting dynamic invoke in F#. We can use it to write code that looks almost like an ordinary method call or property access, but is resolved dynamically at runtime (using the name of the method or property). The following example shows what we'll be able to write at the end of this article:
// Call 'GetProducts' procedure with 'CategoryID' set to 1
use conn = new DynamicSqlConnection(connectionString)
use cmd = conn?GetProducts
cmd?CategoryID <- 1
conn.Open()
// Read all products and print their names
use reader = cmd.ExecuteReader()
while reader.Read() do
printfn "Product: %s" reader?ProductName
If you ever tried to call a SQL stored procedure directly using the
SqlCommand
, then you can surely appreciate the elegance of this code
snippet. Let's now take a look at a larger example and some of the neat
tricks that make this possible...
Published: Friday, 9 July 2010, 2:03 AM
Tags:
functional, web, f#
Read the complete article
ASP.NET and F# (I.) - Creating MVC web applications in F#
Some time ago, I wrote a couple of examples of developing web applications in F# using ASP.NET. Since then, the F# language and runtime has changed a little bit and there are also new technologies available in ASP.NET, so I thought I'd write a more up-to-date article on this topic. In this article, I'll present a simple "demo" F# web application that you can use as a starting point when creating your own projects (you'll also find a convenient Visual Studio 2010 template below). The article shows the following interesting things:
- ASP.NET MVC - We're going to use ASP.NET MVC Framework to create the web application. As the article name suggests, most of the actual program code including models and controllers will be implemented in F#.
- F# LINQ to SQL - The application uses a sample Northwind database and we'll write queries for selecting data from the database using LINQ support that's available in the F# PowerPack.
- F# features - The application also uses some nice F# features that are quite useful for developing web applications. We'll use modules and records to implement the model and we'll also use advanced meta-programming features for constructing LINQ queries.
If you want to use F# for creating an MVC application, you have a few options. It should be possible to create the web application solely as an F# project. However, we'll use a more convenient approach. We'll create a standard C# MVC project and move all the actual implementation to an F# library. We'll look at the application structure shortly. The following screenshot shows a page that lists products in the Northwind database:
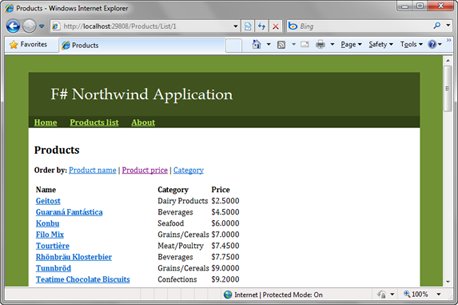
Published: Sunday, 9 May 2010, 8:43 PM
Tags:
.net, functional, web, meta-programming, f#
Read the complete article
Functional Programming in .NET book - An update
Recently, I announced on my blog that I’m working on a book for Manning called Real world Functional Programming in .NET. The goal of the book is to explain the most interesting and useful ideas of functional programming to a real world C# developer. I'm writing this book, because I believe that functional programming is becoming increasingly important. Here is a couple of reasons why you should have this book on your bookshelf:
- Ideas behind C# 3.0 and LINQ - these main-stream technologies are inspired by functional programming and the new C# 3.0 features give us definitely much more than just a new way to query databases. The book explains the ideas behind these features and shows how to use them more efficiently.
- Learning the F# language - F# is becoming a first-class citizen in the Visual Studio family of languages, which alone would be a good reason for learning it! Even if you're not going to use it for your next large .NET project, you'll find it useful for quick prototyping of ideas and testing how .NET libraries work thanks to the great interactive tools.
- Real world examples - the book includes a large set of real-world examples that show how to develop real applications in a functional way - both in F# and C#. Among other things, the examples show how to utilize multi-core CPUs, how to better obtain and process data and how to implement animations and GUI applications in a functional way.
The book is available via the MEAP (Manning Early Access Program) and if you want to get a better idea what is the book about, you can read the first chapter for free. Anyway, it is more than a month since I posted the announcement, so I decided to write a brief update....
Published: Monday, 20 October 2008, 10:10 PM
Tags:
functional, c#, universe, web, writing
Read the complete article
Thesis: Client-side Scripting using Meta-programming
I realized that I haven’t yet posted a link to my Bachelor Thesis, which I partially worked on during my visit in Microsoft Research and which I successfully defended last year. The thesis is about a client/server web framework for F# called F# WebTools, which I already mentioned here and its abstract is following:
“Ajax” programming is becoming a de-facto standard for certain types of web applications, but unfortunately developing this kind of application is a difficult task. Developers have to deal with problems like a language impedance mismatch, limited execution runtime in web browser on the client-side and no integration between client and server-side parts that are developed as a two independent applications, but typically form a single and homogenous application. In this work we present the first project that deals with all three mentioned problems but which still integrates with existing web technologies such as ASP.NET on the server and JavaScript on the client. We use the F# language for writing both client and server-side part of the web application, which makes it possible to develop client-side code in a type-safe programming language using a subset of the F# library, and we provide a way to write both server-side and client-side code as a part of single homogeneous type defining the web page logic. The code is executed heterogeneously, part as JavaScript on the client, and part as native code on the server. Finally we use monadic syntax for the separation of client and server-side code, tracking this separation through the F# type system.
The full text is available here: Client side scripting using meta-programming (PDF, 1.31MB)
Published: Monday, 17 March 2008, 10:07 AM
Tags:
random thoughts, universe, web, links
Read the complete article
F# Support for ASP.NET and Notes on Samples
As I mentioned earlier, I spent three months as an intern in Microsoft Research in Cambridge last year and I was working with Don Syme and James Margetson from the F# team. Most of the time I was working on the F# Web Toolkit, which I introduced on the blog some time ago [1], but I also worked on a few additions that are now part of the F# release. Probably the most useful addition is a new implementation of the CodeDOM provider for the F# language which makes it possible to use ASP.NET smoothly from F# (but it can be used in some other scenarios as well) together with two ASP.NET sample applications that you can explore and use as a basis for your web sites. This was actually a part of the distribution for a few months now (I of course wanted to write this article much earlier...), so you may have already noticed, but anyway, I'd still like to write down a short description of these ASP.NET samples and also a few tips for those who're interested in writing web applications in F#.
Published: Saturday, 8 March 2008, 11:07 PM
Tags:
web, f#
Read the complete article
F# Web Tools: "Ajax" applications made simple
Traditional "Ajax" application consists of the server-side code and the client-side part written in JavaScript (the more dynamicity you want, the larger JS files you have to write), which exchanges some data with the server-side code using XmlHttpRequest, typically in JSON format. I think this approach has 3 main problems, which we tried to solve in F# Web Toolkit. There are a few projects that try to solve some of them already - the most interesting projects are Volta from Microsoft [1], Links language [3] from the University of Edinburgh and Google Web Toolkit [2], but none of the projects solve all three problems at once.
- Limited client-side environment
- Discontinuity between server and client side
- Components in web frameworks are only server-side
The aim of the F# Web Toolkit is to solve all these three problems...
Published: Friday, 13 July 2007, 4:32 AM
Tags:
web, f#
Read the complete article
ASP.NET web applications in F#
CodeDOM (Code Document Object Model) is set of objects (located in System.CodeDom
namespace) that can be used
for representing logical structure of .NET source code. These classes are used for generating Web service references
(using wsdl.exe
tool), for generating typed datasets and in many other situations. The most interesting
use of CodeDOM classes is in ASP.NET where ASP.NET generates code from aspx
/ascx
files and
compiles this code into web site assemblies (together with the code written in code behind files).
This means, that you can use any language for developing ASP.NET web sites, as long as you implement CodeDOM provider
that generates source code from CodeDOM structure and can compile these source files (this can be simply done by executing
command line compiler).
I was recently working on CodeProvider for the F# language, and finally it supports everything
what is needed by ASP.NET (however it is complete and it doesn't work for example with wsdl.exe
). Using this
CodeDOM provider you can write ENTIRE web site in F# (including in-line code enclosed in <% ... source code ... %>).
I also created project template for F# web site that can be imported to Visual Studio 2005, so you can easilly try
writing web pages in F#...
Published: Sunday, 13 August 2006, 9:06 PM
Tags:
web, f#
Read the complete article